JavaScript does a lot of the work behind interactive web pages, from animations to data changes. But what kind of magic is that? To keep your website going smoothly, think of a crew working hard behind the scenes to organize tasks and keep track of resources. This is what JavaScript's Call Stack and Memory Heap do on the inside. These are two very important systems that handle memory and run tasks.
This article goes into detail about these parts, explaining how they work and why JavaScript writers need them. Let's take a look at these ideas one by one, with new ideas and cases you might not have seen before.
Call Stack: How JavaScript Manages Execution
Definition:
The Call Stack is a type of data structure that keeps track of the processes that are currently running in a program. Because JavaScript only uses one thread, it has one Call Stack that it uses to keep track of function calls and the order in which they are run. Because JavaScript is single-threaded, it can only do one thing at a time. For smooth performance, it is important to handle the stack well.
Internal Mechanics of the Call Stack
At its core, the Call Stack works with two primary operations:
- Push – Adds a function to the top of the stack when a function is called.
- Pop – Removes a function from the top of the stack when the function completes or returns.
Detailed Step-by-Step Example:
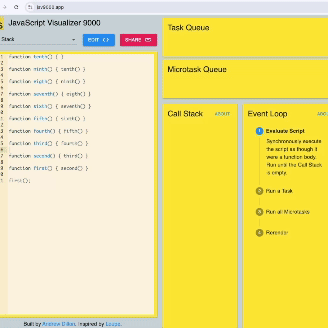
Explanation of LIFO in This Example
- Here’s how the Call Stack manages this code execution:
- Calling
first()
:first()
is invoked.first()
is pushed onto the stack as it’s now the active context.
- Inside
first
, Callingsecond()
:second()
is called from withinfirst()
.second()
is pushed onto the stack, and it’s now at the top, holding control.
- Inside
second
, Callingthird()
:third()
is called from withinsecond()
.third()
is pushed onto the stack, becoming the topmost frame in the stack.
- Executing
third()
:third()
runs and logs “Hello from thirdFunction.”- After it completes,
third()
is popped from the stack.
- Returning to
second()
:- With
third()
done tillTenth
, control goes back tofirst()
.
- With
- Returning to
firstFunction()
:- Finally,
firstFunction()
resumes, completes, and is popped from the stack, leaving the stack empty.
- Finally,
By running this code in JavaScript Visualizer 9000, you’ll see each function call layered onto the stack, with each function removed in reverse order, showcasing the LIFO behavior effectively.
Understanding Execution Contexts
Each item in the Call Stack is an execution context. When a function is invoked, JavaScript creates an execution context containing:
- Variable Environment: Stores local variables and parameters specific to that function.
- Lexical Environment: References the outer scope that the function can access, which forms the scope chain.
- This Binding: Refers to the
this
keyword within that function.
The processing context of each function is important for keeping track of its state and scope while it's running on the stack. This lets JavaScript handle function calls inside functions and return control properly.
Call Stack Frame Structure
Each function call in the stack is represented by a stack frame:
- Function Reference: Points to the function being executed.
- Arguments: Contains the arguments passed to the function.
- Return Address: Keeps track of where to return control after the function completes.
- Local Variables: Stores all local variables declared within the function.
As each function runs, it generates a new stack frame, and as it completes, this frame is removed.
Stack Overflow: Exceeding Stack Capacity
The Call Stack is given a set amount of memory by JavaScript systems. A stack overflow happens when functions keep adding to the stack without finishing, which can happen because of infinite loops or too much nesting.
function infiniteRecursion() {
infiniteRecursion();
}
infiniteRecursion();
In this example:
infiniteRecursion()
calls itself indefinitely, continuously adding new frames to the stack without any exits.- Eventually, the stack memory limit is reached, causing a
RangeError: Maximum call stack size exceeded
.
Most JavaScript engines (like V8 in Chrome) impose a specific Call Stack depth limit to prevent this. This limit can vary across environments and is generally higher in server environments (Node.js) than in browser environments.
Event Loop Interaction
The Call Stack works closely with the Event Loop in JavaScript:
- When a function is called, it’s placed on the Call Stack.
- If a function triggers asynchronous operations (e.g.,
setTimeout
, promises, AJAX requests), these are moved to the Web APIs and don’t directly enter the stack. - Once the asynchronous operation is complete, it’s queued for execution.
- The Event Loop checks if the Call Stack is empty; if so, it pushes the queued task onto the stack.
This interaction allows JavaScript to handle asynchronous operations while maintaining a single-threaded Call Stack.
Call Stack and Error Handling
In JavaScript errors, there is often a stack trace that shows the list of function calls that led to the mistake. This trace shows what was in the Call Stack at the time of the mistake, which helps you figure out what went wrong.
Example:
function first() {
second();
}
function second() {
third();
}
function third() {
throw new Error("An error occurred");
}
first();
When third()
throws an error:
- JavaScript provides a stack trace showing that
third()
was called bysecond()
, which was called byfirst()
. - This trace helps developers identify the error’s origin and call sequence.
Memory management: Role of Garbage collection
To fully understand how Garbage Collection in JavaScript works, let's take a look at how memory is allocated and freed, as well as the tools the garbage collector uses to keep track of memory. To keep applications running smoothly and stop memory leaks, which can slow them down or even crash them over time, you need to handle memory well.
What Is Garbage Collection?
GC, or Garbage Collection, is how JavaScript gets rid of memory that is no longer being used by some other program. In JavaScript, writers don't have to manually give and take away memory; the JavaScript engine does it for them. This process is mostly about finding memory items that can't be reached and taking back the space they take up.
Key Concepts in Garbage Collection
- Memory Allocation: When variables, objects, or functions are created, memory is allocated in the Memory Heap—an area dedicated to storing dynamic data.
- Memory Reclamation: When data is no longer needed, the garbage collector reclaims that memory, freeing it for future allocations.
- Reachability: The main criterion for determining if memory can be reclaimed is reachability—whether or not a piece of data is accessible from the root of the program’s execution.
How Does JavaScript Garbage Collection Work?
JavaScript engines like V8 (used by Chrome and Node.js) primarily use a Mark-and-Sweep algorithm for garbage collection. Here’s a detailed breakdown of how it works:
1. Mark-and-Sweep Algorithm
The Mark-and-Sweep algorithm is the most widely used garbage collection technique in JavaScript. Here’s how it operates:
- Mark Phase:
- The garbage collector begins at the root (typically the global
window
orglobal
object) and “marks” all accessible objects. - It recursively follows references from each accessible object, marking every object it encounters as “reachable.”
- The garbage collector begins at the root (typically the global
- Sweep Phase:
- After marking all reachable objects, the garbage collector sweeps through memory, identifying any unmarked objects.
- Unmarked (or unreachable) objects are those that are not referenced by any other object in memory, making them eligible for deletion.
- The memory occupied by these unmarked objects is then reclaimed.
Example of Reachable and Unreachable Objects
function createData() {
let user = { name: "John" }; // user is reachable
let tempData = { temp: 123 }; // tempData is reachable only within createData
}
createData();
// After createData completes, tempData is unreachable and can be garbage collected
In this example:
user
is a local object within the functioncreateData
.- Once
createData
completes, there are no references totempData
, making it unreachable. The garbage collector will eventually reclaim the memory fortempData
as it’s no longer needed.
2. Generational Garbage Collection
Modern JavaScript engines, such as V8, also use Generational Garbage Collection to improve efficiency. In this model, objects are categorized into two generations:
- Young Generation: Short-lived objects, such as temporary variables, are placed here. These objects are frequently checked and cleared.
- Old Generation: Longer-lived objects, which survive multiple GC cycles, are moved to the Old Generation, where they are checked less frequently.
Generational GC optimizes performance by focusing on reclaiming memory from short-lived objects, which are typically the majority, thereby reducing the overhead of full memory sweeps.
Reference Counting: Another Approach
Reference Counting is a different way to collect garbage. In this method, each object has a count of references that point to it. If an object's reference count drops to zero, it can be deleted because it can't be reached. But reference counting doesn't work well with cyclic references, which are when two things reference each other and can't be deleted even though they can't be reached.
Example of a Cyclic Reference Issue
function createCycle() {
let objA = {};
let objB = {};
objA.reference = objB;
objB.reference = objA;
}
In this example:
objA
andobjB
reference each other, creating a cycle.- Since reference counts do not reach zero, these objects are retained in memory, causing a memory leak.
Modern JavaScript engines avoid this issue by using Mark-and-Sweep instead of reference counting.
Garbage Collection Trigger Conditions
JavaScript garbage collection isn’t continuous; it’s triggered under specific conditions to balance performance and memory usage:
- Memory Thresholds: GC may run when memory usage exceeds a certain limit.
- Idle Time: In browser environments, GC often runs during idle times, minimizing performance impact.
- Manual Trigger: Some environments, like Node.js, allow manual garbage collection for debugging purposes (using
node --expose-gc
), but this isn’t generally used in production due to performance concerns.
When triggered, GC can perform:
- Minor Collection: Focused on the Young Generation, targeting short-lived objects.
- Major Collection: Sweeps both generations and is less frequent but more comprehensive.
Common Issues with Garbage Collection
Even with automatic garbage collection, developers need to be aware of certain patterns that can lead to memory inefficiencies:
- Memory Leaks:
- Global Variables: Variables declared globally persist throughout the program’s lifecycle, retaining memory.
- Event Listeners: If not properly removed, event listeners on removed DOM elements can retain memory.
- Closures: Closures retain references to the outer scope. Excessive use of closures can lead to memory retention.
- Memory Bloat:
- Large Data Structures: Holding onto large arrays or objects longer than necessary can bloat memory usage.
- Frequent Small Allocations: Repeatedly creating small objects can increase the frequency of garbage collection, impacting performance.
- Performance Slowdowns:
- Major GC cycles can cause noticeable pauses if they run too frequently or at inopportune times. By managing data usage effectively, you can help reduce the frequency and impact of these cycles.
Best Practices for Efficient Memory Management
To work harmoniously with the garbage collector and prevent memory issues, follow these best practices:
- Minimize Global Variables: Use
const
,let
, orvar
within functions or blocks to avoid unnecessary global scope memory. - Detach Event Listeners: Always remove event listeners from DOM elements that are no longer in use.
- Use Weak References: In cases where you need a reference without preventing GC, use
WeakMap
orWeakSet
, as these structures don’t prevent garbage collection. - Avoid Excessive Closures: While closures are powerful, be mindful of excessive use, as they can retain references to variables in their scope.
- Optimize Object and Array Usage: Reuse objects where possible and clear large arrays or objects when they’re no longer needed.
Summary: Memory Management in JavaScript
The Call Stack and Garbage Collection are the two main ways that JavaScript manages memory. Together, they make sure that memory is used efficiently, so programs can run easily without the developer having to do anything.
Call Stack: The Call Stack is a key part of keeping track of the order in which JavaScript functions are run. It uses the Last-In, First-Out (LIFO) method, which means that functions are pushed to the top of the stack as they are called and popped off as they finish. By keeping a single-threaded stack, JavaScript makes sure that tasks run at the same time and helps stop common problems like stack overflow, which happens when too many functions are called in a loop or inside another loop without finishing.
Garbage Collection (GC): GC automatically cleans up memory by finding things that aren't reachable and freeing up memory that they are using. Mark-and-Sweep is the main algorithm used. It marks all items that can be reached and removes those that are no longer being used. Generational Collection is also used by modern engines to better handle things with short and long lives. Garbage Collection frees memory from objects that aren't being used, but some patterns, like cyclic references, global variables, and event listeners that haven't been removed, can still cause memory retention if they aren't handled properly.
In short, if you want to handle memory well in JavaScript, you need to know how the Call Stack organizes function execution and how Garbage Collection gets rid of memory that isn't being used. They work together to automatically assign, track, and free memory, which lowers the risk of memory leaks, stack overflows, and slowdowns. To make fast, stable apps, you need to write code that is efficient and works well with these platforms.
Discussion